C 20Day (20160203)
by jennysgap1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <stdio.h> struct mk_char { char name[30]; int level, str, dex, luk; }; int main(void) { struct mk_char user2={"주먹펴고 일어서", 0, 0, 0, 0}; struct mk_char * pptr=&user2; //구조체 포인터변수를 선언하는 방법 (*pptr).level=100; pptr->level=200; //애로우연산자 (위에랑 같은 의미) printf("%s\n", user2.name); printf("%d\n", user2.level); printf("%d %d %d\n", user2.str, user2.dex, user2.luk); return 0; } | cs |
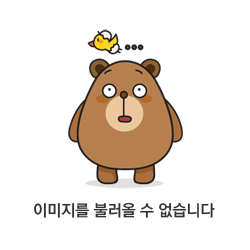
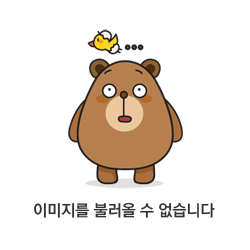
구조체변수와 포인터
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | #include <stdio.h> struct point { int xpos; int ypos; }; int main(void) { struct point pos1={1, 2}; struct point pos2={100, 200}; struct point * pptr=&pos1; (*pptr).xpos += 4; (*pptr).ypos += 5; printf("[%d, %d] \n", pptr->xpos, pptr->ypos); pptr=&pos2; pptr->xpos += 1; pptr->ypos += 2; printf("[%d, %d] \n", (*pptr).xpos, (*pptr).ypos); return 0; } | cs |
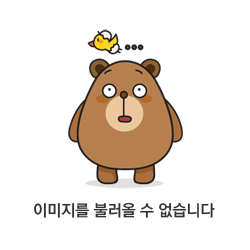
예제2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | #include <stdio.h> struct point { int xpos; int ypos; }; struct circle { double radius; struct point * center; }; int main(void) { struct point cen={2, 7}; double rad=5.5; struct circle ring={rad, &cen}; printf("원의 반지름: %g \n", ring.radius); printf("원의 중심 [%d, %d] \n", (ring.center)->xpos, (ring.center)->ypos); return 0; } | cs |
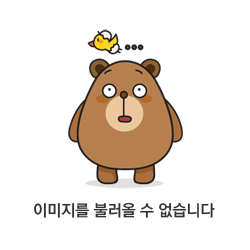
구조체 변수도 배열 가능 할까요? 가능!
동적할당
: 프로그래머가 원하는 시점에 메모리를 할당하고 원하는 시점에 메모리를 소멸시키는 것
: 동적할당엔 이름이 존재하지 않음. (그냥 Heap 부분에 메모리 할당만) So 포인트변수를 이용해서 간접접근
: 동적할당 했으면 반드시 소멸시점도 입력해야함.
malloc( ) : 원하는 시점에 메모리 할당
free( ) : 원하는 시점에 메모리 소멸
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> //malloc 헤더파일 int main(void) { //malloc(크기); //~크기만큼 동적할당 할거야 //void * ptr=malloc(4); 포인터변수로 접근 (자료형을 아직 알 수가 없어 void 입력 but 안됨) //void * ptr=malloc(sizeof(int)); (정상적으로 표인트 변수로 선언 안됨) int * ptr=(int *)malloc(sizeof(int)); //강제형변환! 동적할당을 하기위한 가장 일반적인 방법!!!!!!! *ptr=10; //동적할당 printf("%d\n", *ptr); free(ptr); //동적할당할 경우 반드시 소멸 입력해야한다!!! return 0; } | cs |
예제1
동적할당 실수형태 3.14 입력해보기
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> #include <stdlib.h> int main(void) { double * ptr=(double *)malloc(sizeof(double)); *ptr=3.14; printf("%f \n", *ptr); free(ptr); return 0; } | cs |
예제2
int형 배열처럼 동적할당을 작성하라.
1 2 3 4 5 6 7 8 9 10 11 | #include <stdio.h> #include <stdlib.h> int main(void) { int * ptr=(int *)malloc(sizeof(int)*5); free(ptr); return 0; } | cs |
값 부여
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <stdio.h> #include <stdlib.h> int main(void) { int * ptr=(int *)malloc(sizeof(int)*5); *ptr=10; *(ptr+1)=20; *(ptr+2)=30; *(ptr+3)=40; *(ptr+4)=50; printf("%d ", *ptr); printf("%d ", *(ptr+1)); printf("%d ", *(ptr+2)); printf("%d ", *(ptr+3)); printf("%d ", *(ptr+4)); free(ptr); return 0; } | cs |
반복문으로 간결하게 작성해보기 *(ptr+i) == ptr[i]
1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> int main(void) { int i; int * ptr=(int *)malloc(sizeof(int)*5); for (i=0; i<5; i++) { *(ptr+i)=(i+1)*10; printf("%d ", *(ptr+i)); } free(ptr); return 0; } | cs |
2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> int main(void) { int i; int * ptr=(int *)malloc(sizeof(int)*5); for (i=0; i<5; i++) { ptr[i]=(i+1)*10; printf("%d ", ptr[i]); } free(ptr); return 0; } | cs |
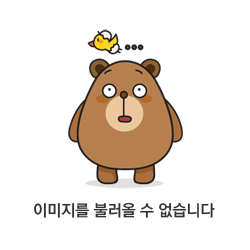
반응형
블로그의 정보
jennysgap
jennysgap